template<class _Advanceable>
AdvanceableRunner class
AdvanceableRunner is an helper class that can be used to run a advanceable at a given period.
Different AdvanceableRunners can communicate trough the SharedResource class. The AdvanceableRunner can be used only with Advanceable. Do not try to use if with a class that does not inherit from advanceable, a compile time assert will be thrown. This is a visual example of usage
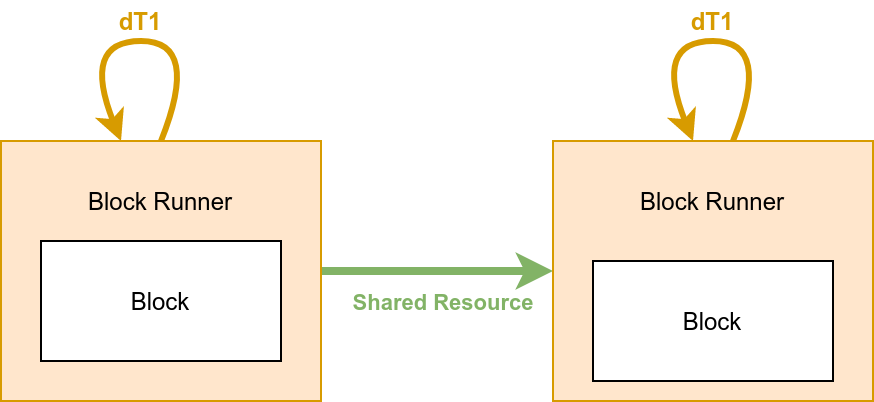
Here we want to run two advanceables in parallel with a different sampling time. The AdvanceableRunner is a class that helps you in creating a periodic thread. The SharedResource is the way that can be used to communicate between two advanceables running in separate threads (i.e. the wired between two AdvanceableRunners)
Public types
Public functions
-
auto initialize(std::weak_ptr<const ParametersHandler::
IParametersHandler> handler) -> bool - Initialize the AdvanceableRunner class.
- auto setAdvanceable(std::unique_ptr<_Advanceable> advanceable) -> bool
- Set the advanceable inside the runner.
- auto setInputResource(std::shared_ptr<SharedResource<Input>> resource) -> bool
- Set the input resource.
- auto setOutputResource(std::shared_ptr<SharedResource<Output>> resource) -> bool
- Set the output resource.
- auto getInfo() -> Info
- Get some info of the runner,.
- auto run(std::optional<std::reference_wrapper<Barrier>> barrier = {}) -> std::thread
- Run the advanceable runner.
- void stop()
- Stop the thread.
- auto isRunning() const -> bool
- Check if the AdvanceableRunner is running.
Function documentation
template<class _Advanceable>
bool BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: initialize(std::weak_ptr<const ParametersHandler:: IParametersHandler> handler)
Initialize the AdvanceableRunner class.
Parameters | |
---|---|
handler | pointer to a parameter handler |
Returns | true in case of success, false otherwise. |
template<class _Advanceable>
bool BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: setAdvanceable(std::unique_ptr<_Advanceable> advanceable)
Set the advanceable inside the runner.
Parameters | |
---|---|
advanceable | an unique pointer representing the advanceable |
Returns | true in case of success, false otherwise |
template<class _Advanceable>
bool BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: setInputResource(std::shared_ptr<SharedResource<Input>> resource)
Set the input resource.
Parameters | |
---|---|
resource | pointer representing the input resource |
Returns | true in case of success, false otherwise |
template<class _Advanceable>
bool BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: setOutputResource(std::shared_ptr<SharedResource<Output>> resource)
Set the output resource.
Parameters | |
---|---|
resource | pointer representing the output resource |
Returns | true in case of success, false otherwise |
template<class _Advanceable>
Info BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: getInfo()
Get some info of the runner,.
Returns | A copy of the Info struct |
---|
template<class _Advanceable>
std::thread BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: run(std::optional<std::reference_wrapper<Barrier>> barrier = {})
Run the advanceable runner.
Parameters | |
---|---|
barrier | is an optional parameter that can be used to synchronize the startup of all the AdvanceableRunner spawned by the process. |
Returns | a thread of containing the running process. If the runner was not correctly initialized the thread is invalid. |
The function create a periodic thread running with a period of m_dT seconds.
template<class _Advanceable>
void BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: stop()
Stop the thread.
Returns | true in case of success, false otherwise |
---|
template<class _Advanceable>
bool BipedalLocomotion:: System:: AdvanceableRunner<_Advanceable>:: isRunning() const
Check if the AdvanceableRunner is running.
Returns | true if the thread is running, false otherwise. |
---|