class
MANNTrajectoryGeneratorMANNTrajectoryGenerator is a class that uses MANNAutoregressive to generate a kinematically feasible trajectory for humanoid robots.
The planner will generate a trajectory which duration is equal to slow_down_factor * time_horizon
seconds.
MANN trajectory generator
The diagram illustrates the utilization of the MANNAutoregressive within the MANNTrajectoryGenerator class.
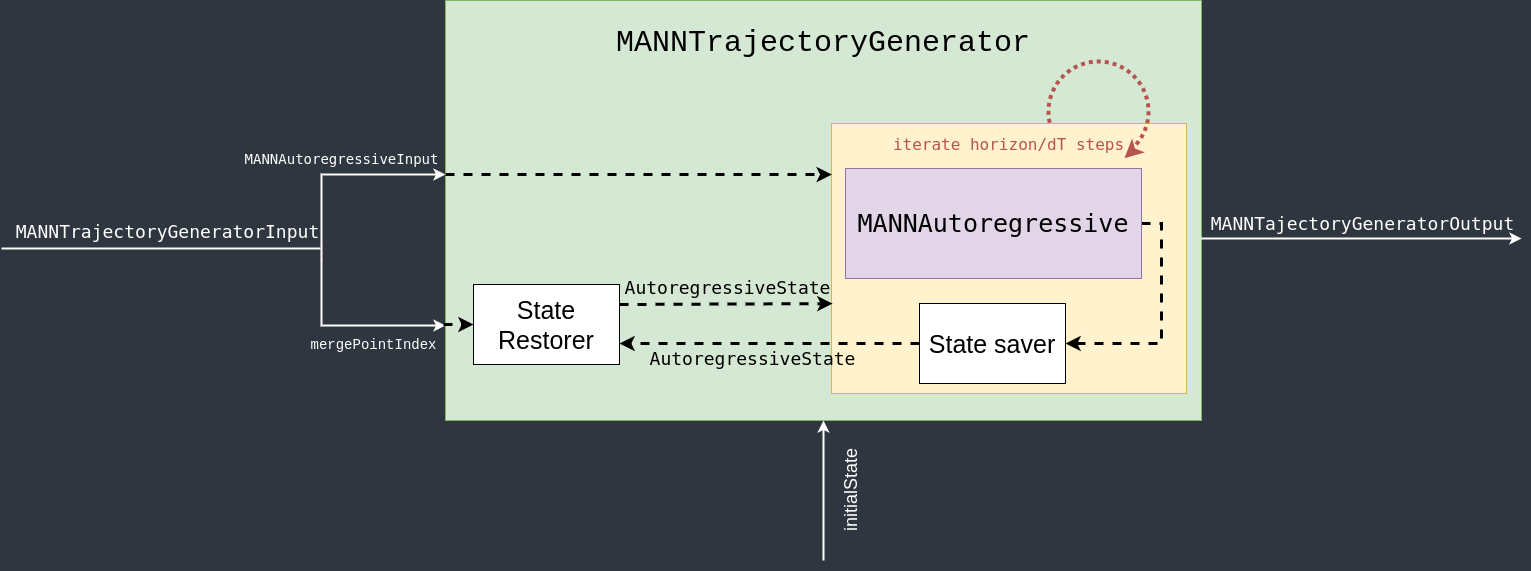
To initialize the generator, the user needs to set the initial state using the MANNTrajectoryGenerator::
Base classes
-
template<class _Input, class _Output>class BipedalLocomotion::System::Advanceable<MANNTrajectoryGeneratorInput, MANNTrajectoryGeneratorOutput>
- Basic class that represents a discrete system.
Constructors, destructors, conversion operators
- MANNTrajectoryGenerator()
- Constructor.
- ~MANNTrajectoryGenerator()
- Destructor.
Public functions
- auto setRobotModel(const iDynTree::Model& model) -> bool
- Set the robot model.
-
auto initialize(std::weak_ptr<const ParametersHandler::
IParametersHandler> paramHandler) -> bool override - Initialize the trajectory planner.
- auto setInput(const Input& input) -> bool override
- Set the input to the planner model.
- auto advance() -> bool override
- Generate the trajectory.
- auto isOutputValid() const -> bool override
- Check if the output is valid.
- auto getOutput() const -> const Output& override
- Get the output from trajectory.
-
void setInitialState(Eigen::Ref<const Eigen::VectorXd> jointPositions,
const Contacts::
EstimatedContact& leftFoot, const Contacts:: EstimatedContact& rightFoot, const manif::SE3d& basePose, const std::chrono::nanoseconds& time) - Set the initial state of the planner.
Function documentation
bool BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: setRobotModel(const iDynTree::Model& model)
Set the robot model.
Parameters | |
---|---|
model | model of the robot considered by the network. Please load the very same model with the same joint serialization used to train the MANN network. |
Returns | true in case of success, false otherwise. |
bool BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: initialize(std::weak_ptr<const ParametersHandler:: IParametersHandler> paramHandler) override
Initialize the trajectory planner.
Parameters | |
---|---|
paramHandler | pointer to the parameters handler. |
Returns | True in case of success, false otherwise. |
bool BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: setInput(const Input& input) override
Set the input to the planner model.
Parameters | |
---|---|
input | input to the model |
Returns | true in case of success, false otherwise. |
bool BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: advance() override
Generate the trajectory.
Returns | true in case of success, false otherwise. |
---|
bool BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: isOutputValid() const override
Check if the output is valid.
Returns | true if the output is valid, false otherwise. |
---|
const Output& BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: getOutput() const override
Get the output from trajectory.
Returns | the output of the system. |
---|
void BipedalLocomotion:: ML:: MANNTrajectoryGenerator:: setInitialState(Eigen::Ref<const Eigen::VectorXd> jointPositions,
const Contacts:: EstimatedContact& leftFoot,
const Contacts:: EstimatedContact& rightFoot,
const manif::SE3d& basePose,
const std::chrono::nanoseconds& time)
Set the initial state of the planner.
Parameters | |
---|---|
jointPositions | position of the joints. |
leftFoot | |
rightFoot | |
basePose | pose of the base. |
time | initial time of the planner. |